Community Project Collection: How to Input data to Wio Terminal from a Keyboard Using USB Host Function, and Tips about How to Use the 40-Pin Header for Power Supply and USB Serial
This tutorial is translated by Seeed from Wio Terminal でUSB Host機能を堪能 written by homemadegarbage (twitter: @H0meMadeGarbage). Thank you for sharing this with us! Visit their homepage for more interesting projects: homemadegarbage.com.
Wio Terminal is equipped with USB Host function and can be connected to USB Device. In this demo, a keyboard is connected to Wio Terminal for data input. As shown on the LCD screen, different keys are assigned with different music scales and can play a melody together.
This post will be covered by:
- 1. Install USB Host Library SAMD
- 2. Connect the Keyboard
- 3. Sample Code
- 4. Debug
1. Install USB Host Library SAMD
To use the USB Host on Wio Terminal, USB Host Library SAMD is essential. Check here to learn about how to install it.
2. Connect the Keyboard
Refer to this tutorial and connect the keyboard to Wio Terminal. You will also need a USB-C OTG cable.
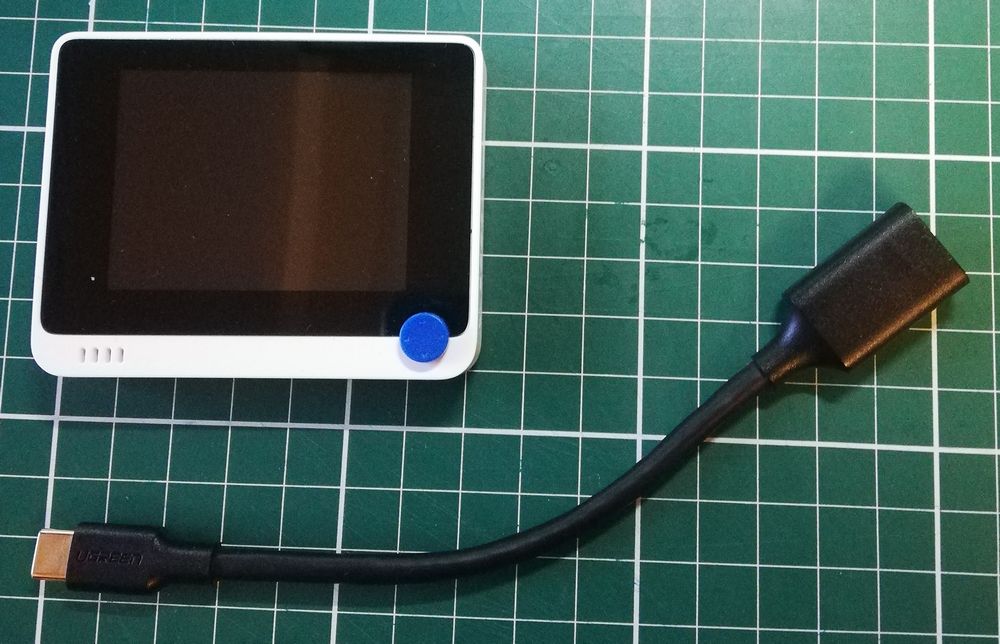
When using the USB Host function, power supply and USB serial communication cannot be used since the USB Type-C interface has been taken, so we will use the 40-pin header on the back of Wio Terminal instead.
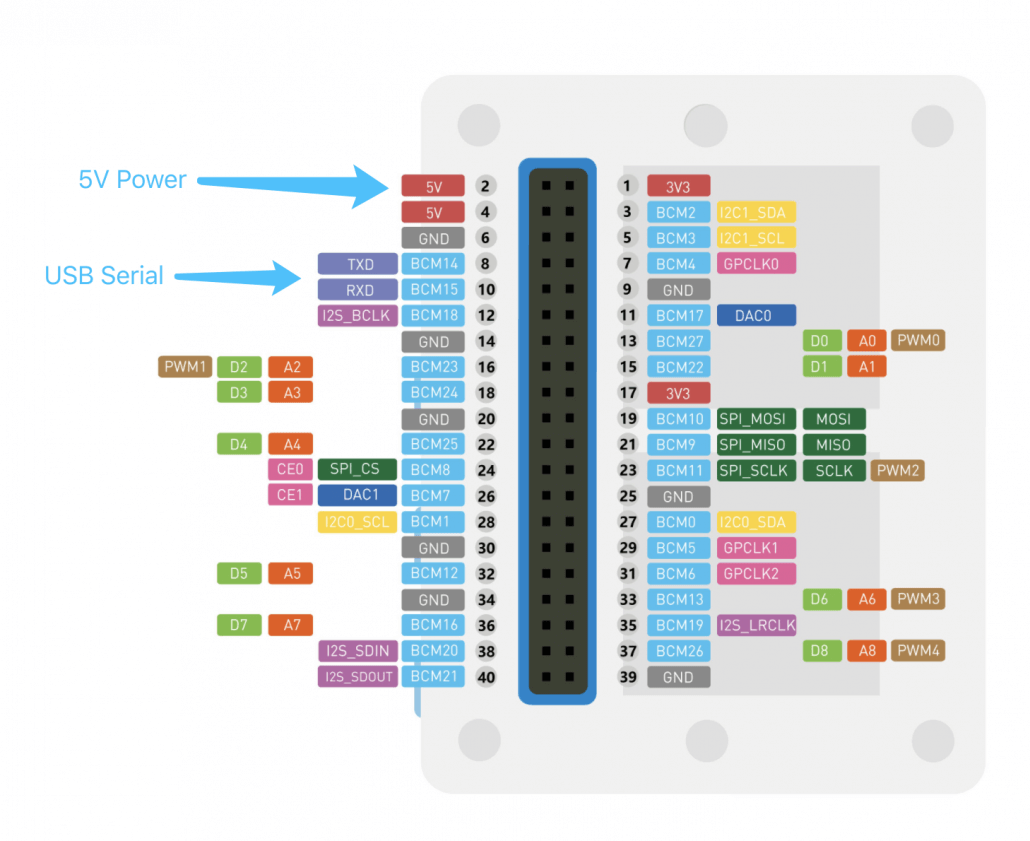
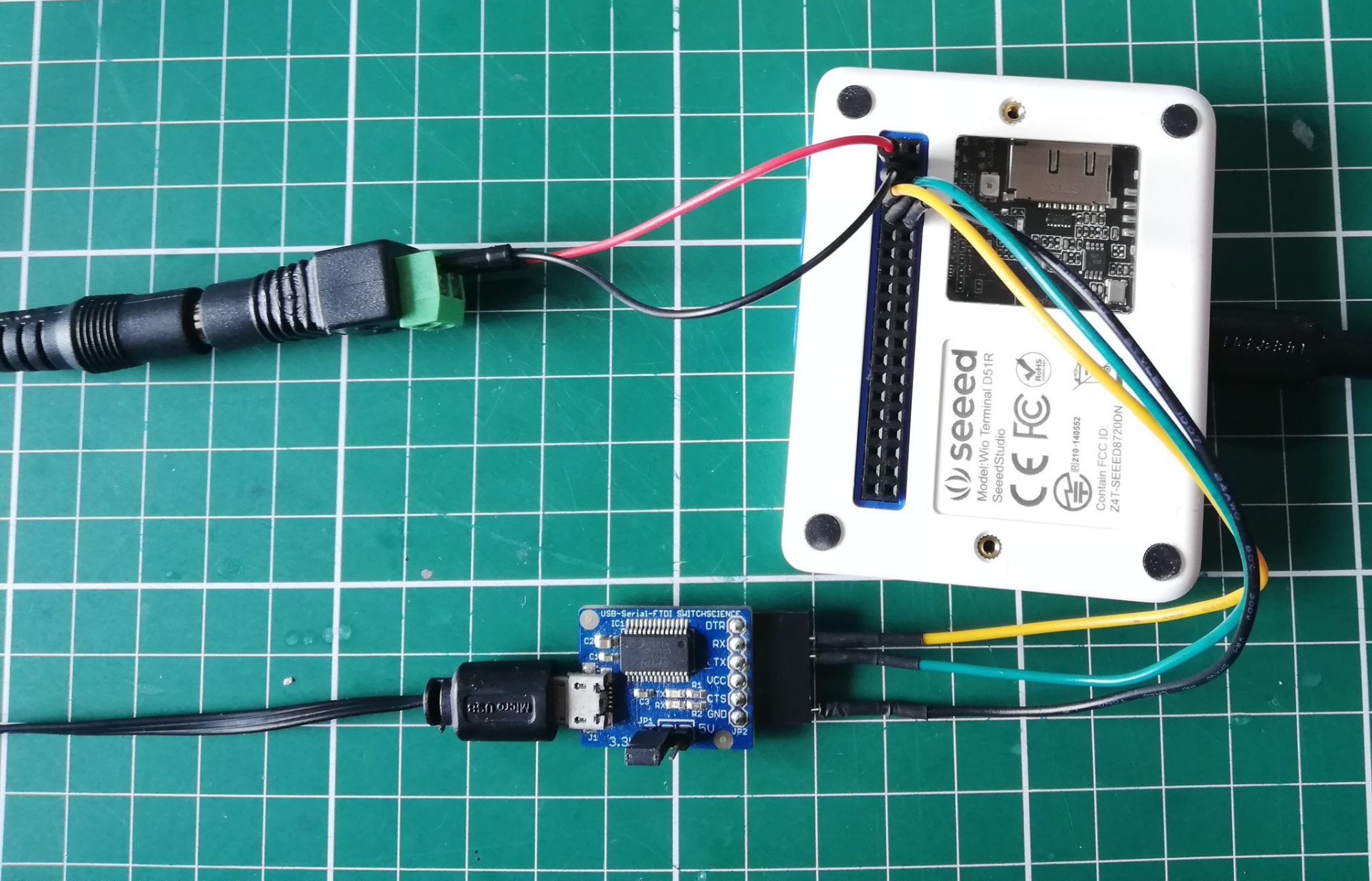
The power supply of the USB serial conversion cable is set to 3.3V (The FTDI USB to Serial Adapter used in this demo can be found on Amazon).
After that, it can be output with Serial1 and monitored with some common serial communication software such as Tera Term.
3. Sample Code
Reference:
https://wiki.seeedstudio.com/Wio-Terminal-USBH-Keyboard/
https://wiki.seeedstudio.com/Wio-Terminal-LCD-Basic/
https://wiki.seeedstudio.com/Wio-Terminal-Buzzer/
#include <KeyboardController.h>
#define SerialDebug Serial1
#include"TFT_eSPI.h"
#include"Free_Fonts.h" //include the header file
#define BUZZER_PIN WIO_BUZZER
// Initialize USB Controller
USBHost usb;
// Attach keyboard controller to USB
KeyboardController keyboard(usb);
void printKey();
TFT_eSPI tft;
int key;
// This function intercepts key press
void keyPressed() {
SerialDebug.print("Pressed: ");
printKey();
switch (key) {
case 20:
tft.drawString("C",140,100);
playTone(1915, 400);
break;
case 26:
tft.drawString("D",140,100);
playTone(1700, 400);
break;
case 8:
tft.drawString("E",140,100);
playTone(1519, 400);
break;
case 21:
tft.drawString("F",140,100);
playTone(1432, 400);
break;
case 23:
tft.drawString("G",140,100);
playTone(1275, 400);
break;
case 28:
tft.drawString("A",140,100);
playTone(1136, 400);
break;
case 24:
tft.drawString("B",140,100);
playTone(1014, 400);
break;
case 12:
tft.drawString("C+",140,100);
playTone(956, 400);
break;
}
}
// This function intercepts key release
void keyReleased() {
SerialDebug.print("Released: ");
printKey();
tft.fillScreen(TFT_BLACK);
digitalWrite(BUZZER_PIN, LOW);
}
void printKey() {
// getOemKey() returns the OEM-code associated with the key
key = keyboard.getOemKey();
SerialDebug.print(" key:");
SerialDebug.print(key);
// getModifiers() returns a bits field with the modifiers-keys
int mod = keyboard.getModifiers();
SerialDebug.print(" mod:");
SerialDebug.print(mod);
SerialDebug.print(" => ");
if (mod & LeftCtrl)
SerialDebug.print("L-Ctrl ");
if (mod & LeftShift)
SerialDebug.print("L-Shift ");
if (mod & Alt)
SerialDebug.print("Alt ");
if (mod & LeftCmd)
SerialDebug.print("L-Cmd ");
if (mod & RightCtrl)
SerialDebug.print("R-Ctrl ");
if (mod & RightShift)
SerialDebug.print("R-Shift ");
if (mod & AltGr)
SerialDebug.print("AltGr ");
if (mod & RightCmd)
SerialDebug.print("R-Cmd ");
// getKey() returns the ASCII translation of OEM key
// combined with modifiers.
SerialDebug.write(keyboard.getKey());
SerialDebug.println();
}
uint32_t lastUSBstate = 0;
void setup(){
tft.begin();
tft.setRotation(3);
tft.fillScreen(TFT_BLACK);
tft.setFreeFont(FSSBO24);
SerialDebug.begin( 115200 );
SerialDebug.println("Keyboard Controller Program started");
if (usb.Init())
SerialDebug.println("USB host did not start.");
delay( 20 );
//Coqnfigure pins to enable USB Host on Wio Terminal
digitalWrite(PIN_USB_HOST_ENABLE, LOW);
digitalWrite(OUTPUT_CTR_5V, HIGH);
pinMode(BUZZER_PIN, OUTPUT);
}
void loop()
{
// Process USB tasks
usb.Task();
uint32_t currentUSBstate = usb.getUsbTaskState();
if (lastUSBstate != currentUSBstate) {
SerialDebug.print("USB state changed: 0x");
SerialDebug.print(lastUSBstate, HEX);
SerialDebug.print(" -> 0x");
SerialDebug.println(currentUSBstate, HEX);
switch (currentUSBstate) {
case USB_ATTACHED_SUBSTATE_SETTLE: SerialDebug.println("Device Attached"); break;
case USB_DETACHED_SUBSTATE_WAIT_FOR_DEVICE: SerialDebug.println("Detached, waiting for Device"); break;
case USB_ATTACHED_SUBSTATE_RESET_DEVICE: SerialDebug.println("Resetting Device"); break;
case USB_ATTACHED_SUBSTATE_WAIT_RESET_COMPLETE: SerialDebug.println("Reset complete"); break;
case USB_STATE_CONFIGURING: SerialDebug.println("USB Configuring"); break;
case USB_STATE_RUNNING: SerialDebug.println("USB Running"); break;
}
lastUSBstate = currentUSBstate;
}
}
void playTone(int tone, int duration) {
for (long i = 0; i < duration * 1000L; i += tone * 2) {
digitalWrite(BUZZER_PIN, HIGH);
delayMicroseconds(tone);
digitalWrite(BUZZER_PIN, LOW);
delayMicroseconds(tone);
}
}
4. Debug
After uploading the USB Host code, Wio Terminal will not be recognized even if you connect the Wio Terminal to the PC through its USB-C connector.
In that case, please slide the power button on the side of Wio Terminal twice quickly to put it in the rewrite mode.