Getting started with Arduino Data Types
Arduino data types. They play an important role when it comes to programming the Arduino. The Arduino which is a computer is highly data agnostic (it does not know or care in what manner the data it receives was sent to it.)
Without data types, you cannot determine how many bytes of memory are dedicated to that variable, and what kind of data can be stored in the variable which makes data type of the variable important.
A data type can be defined as a classification that describes the value a variable holds and the operations we can perform on it. Other than variables, functions also have data types depending on the values they return.
In this tutorial, you will learn about all the different data types that you will use when programming your Arduino. The data types are:
- void
- boolean
- char
- Unsigned char
- byte
- int
- Unsigned int
- Word
- Long
- Unsigned long
- short
- float
- double
This tutorial will NOT cover arrays, pointers, or strings as these are more specialized with more underlying concepts.
Without further ado, let us jump right into our first data type, void.
void
void is only used when declaring functions. It is used to indicate that the function is expected to return no values when they are called.
It is important to note that Setup and Loop functions are of type void and do not return any information as well.
Example void code
void setup()
{
Serial.begin(9600);
}
void loop()
boolean
A boolean holds either one of two boolean values, true or false. boolean is a non-standard type alias for bool
defined by Arduino.
This Arduino Data type has a memory of 8 bit / 1 byte.
Example boolean code
int LEDpin = 5; // LED on pin 5
int switchPin = 13; // momentary switch on 13, other side connected to ground
boolean running = false;
void setup()
{
pinMode(LEDpin, OUTPUT);
pinMode(switchPin, INPUT);
digitalWrite(switchPin, HIGH); // turn on pullup resistor
}
void loop()
{
if (digitalRead(switchPin) == LOW)
{ // switch is pressed - pullup keeps pin high normally
delay(100); // delay to debounce switch
running = !running; // toggle running variable
digitalWrite(LEDpin, running); // indicate via LED
}
}
char
char which is short for character, is a data type used to store a character value (A,B,C). When initializing multiple characters it will be written in single quotes (eg. ‘A’) but for strings, they use double quotes (eg.”ABC”)
Characters will be stored as numbers. For the specific encoding, you can refer to the ASCII Chart as shown below.
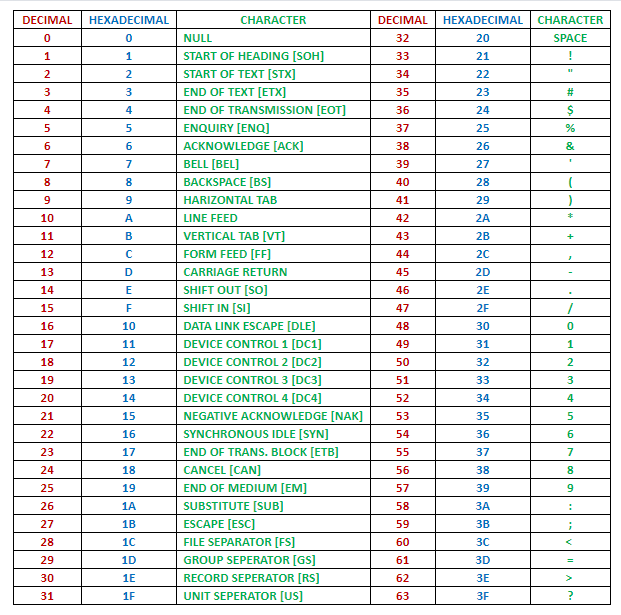
As characters will be stored as numbers, you can do arithmetic operations on them using the ASCII value of the character. The char data type encodes numbers from -128 to 127.
This Arduino data type has a memory of at least 8 bits. You are recommended to use char for storing characters.
Example char code
char myChar = 'A';
char myChar = 65; // both are equivalent
Unsigned char
The unsigned char datatype encodes numbers from 0 to 255.
This Arduino data type has a memory of 8 bit/ 1 byte which is similar to the byte datatype. For clarity and consistency of the Arduino programming style, for an unsigned, one-byte data type, the byte data type is recommended.
Example Unsigned char code
unsigned char myChar = 240;
byte
Similar to the unsigned char data type, a byte encodes an 8-bit unsigned number from 0-255
Example byte code
byte m = 25 ;//declaration of variable with type byte and initialize it with 25
int
int which is short for integer is one of the most commonly used data type in Arduino. They are your primary data type for storing numbers.
Do note that int size varies from board to board. For example, in ATmega based Arduino boards like the Uno, Mega and Nano, an int uses 2 byte of memory and as a range of -32,768 to +32,767. While for the Due and SAMD based boards (eg. MKR1000, Zero), int uses 4 byte of memory and as a range of -2,147,483,648 to + 2,147,483,647.
Example int code
int countUp = 0; //creates a variable integer called 'countUp'
Unsigned int
The unsigned int is similar to int in the way that they store a 2-byte value. However, instead of storing negative numbers, they store only positive values with a range of 0 to +65,535.
Same as int, unsigned int size varies from board to board with ATmega based Arduino boards storing a 2-byte value while the Due and SAMD based boards stores a 4 bytes (32-bit) value and has a range of 0 to 4,294,967,295.
The main difference between unsigned int and ints is how the highest bit/sign bit is interpreted. In the int type (which is signed), if the highest bit is “1”, the number is interpreted as a negative number, and the other 15 bits are interpreted with.
Example unsigned int code
unsigned int ledPin = 13;
Word
The word data type is very similar to the previous unsigned int data type. On the ATmega based Arduino boards, a word stores a 16-bit unsigned number with a 2-byte value and a range from 0 to +65535. As for Due and SAMD based boards, it stores a 32-bit unsigned number with a 4-byte value.
Example Word code
word w = 10000;
Long
Long variables are extended size variables for number storage. Long variables use 4 bytes from memory (32 bits) with a range from -2,147,483,648 to +2,147,483,647.
Example Long code
long speedOfLight = 186000L; //declaration of variable with type Long and initialize it with 186000
Unsigned long
Similar to the Long data type, unsigned long variables are extended size variables for number storage and use 4 bytes from memory (32 bits). However, unlike standard Longs, unsigned longs do not store negative numbers. They have a range of 0 to +4,294,967,295.
Example Unsigned long code
unsigned long time;
void setup() {
Serial.begin(9600);
}
void loop() {
Serial.print("Time: ");
time = millis();
//prints time since program started
Serial.println(time);
// wait a second so as not to send massive amounts of data
delay(1000);
}
short
A short datatype stores a 16 bit value and uses 2 bytes from memory on ALL Arduinos. They have a range of -32,768 to +32,767.
Example short code
short ledPin = 13
float
The float is one of the most important Arduino data type as it can store decimal numbers. This data type is for floating-point numbers which are numbers with a decimal point.
Floating-point numbers are often used to approximate the analog and continuous values because they have greater resolution than integers.
This data type has a memory of 32 bit/ 4 bytes and a range of -3.4028235E+38 to +3.4028235E+38.
Example float code
float myfloat;
float sensorCalbrate = 1.117;
int x;
int y;
float z;
x = 1;
y = x / 2; // y now contains 0, ints can't hold fractions
z = (float)x / 2.0; // z now contains .5 (you have to use 2.0, not 2)
double
This data type is a double-precision floating point number. On ATmega based Arduino boards like the Uno, Mega and Nano, double precision floating-point number occupies 4 bytes (32 bit). That is, the double implementation is exactly the same as the float, with no gain in precision.
While for Due and SAMD based boards (eg. MKR1000, Zero), double have 8 bytes (64-bit) precision.
Example double code
double num = 45.352 ;// declaration of variable with type double and initialize it with 45.352
Which data type do you choose to use when programming your Arduino?
Generally, the data type you choose is not a very big deal during programming. However, when size and speed is concerned, choosing the right data type is crucial.
When picking a data type, try to pick the smallest data type that will fully contain the value you need to store. For example, you want to store a pin number, but your board has less than 128 pins, thus, storing this value in a byte or char type is the best option.
On the other hand, if you have a board with more than 128 pins but less than 255 pins, you can use the byte or unsigned char data type.
By using smaller data types, it can enhance your speed as they require less memory reads.
All in all when choosing your data type, always use the smallest data type necessary to store the value.
Conclusion
That is all for this tutorial. If you have any questions about these Arduino data types, do leave a comment down below!
Want to get started programming your Arduino but do not know which Arduino board to get? No fear as we have a simple table shown below comparing almost all of Arduino models including our very Seeeduino models too.
We listed specifications including processor, operating voltage, input voltage, clock speed, digital I/O, PWM, analog inputs, UART, Grove connectors, flash memory, and USB connectors for 7 Arduino official boards and 13 Seeeduino boards. I hope this table will help you quickly compare different Arduino boards and get one that best fits your project needs.
Processor | Operating Voltage | Clock Speed | Digital I/O | PWM | Analog Inputs | UART | Grove | Flash(KB) | USB | |
---|---|---|---|---|---|---|---|---|---|---|
Arduino Uno | ATmgea328 | 5V | 16 MHZ | 14 | 6 | 6 | 1 | 0 | 32 | Micro USB |
Arduino Mega 2560 R3 | ATmega2560 | 5V | 16 MHZ | 54 | 15 | 16 | 4 | 0 | 256 | Type-B USB |
Arduino Nano | ATmega328 | 5V | 16 MHZ | 14 | 6 | 8 | 1 | 0 | 32 | Mini USB |
Arduino Micro | ATmega32u4 | 5V | 16 MHZ | 20 | 7 | 12 | 2 | 0 | 32 | Micro USB |
Arduino YUN | ATmega32u4 | 5V | 16 MHZ | 20 | 7 | 12 | 2 | 0 | 32 | Micro USB |
Arduino MKR1000 wifi | ATSAMW25 SoC | 5V | 48 MHZ | 8 | 12 | 7 | 1 | 0 | 256 | Micro USB |
Seeeduino Mega | ATmega2560 | 5V/3.3V | 16MHz | 70 | 14 | 16 | 4 | 0 | 256 | Micro USB |
Seeeduino Lite | ATmega32u4 | 5V/3.3V | 16MHz | 20 | 7 | 12 | 1 | 2 | 32 | Micro USB |
Seeeduino GPRS | ATmega32u4 | 5V/3.3V | 16MHz | 20 | 7 | 12 | 1 | 0 | 32 | Micro USB |
Seeeduino Cloud | ATmega32u4 | 5V/3.3V | 16MHz | 20 | 7 | 12 | 1 | 2 | 32 | Micro USB |
Seeeduino V4.2 | ATmega328 | 5V/3.3V | 16MHz | 14 | 6 | 6 | 1 | 3 | 32 | Micro USB |
Seeeduino Stalker V3.1 | Atmega328P | 5V/3.3V | 8MHz | 14 | 0 | 6 | 1 | 2 | 32 | Mini USB |
Seeeduino LoRaWAN | ATSAMD21G18 | 5V/3.3V | 48MHz | 20 | 20 | 6 | 2 | 4 | 256 | Micro USB |
Seeeduino LoRaWAN W/GPS | ATSAMD21G18 | 3.3V | 48MHz | 20 | 20 | 6 | 2 | 4 | 256 | Micro USB |
Seeeduino Lotus V1.1 | Atmega328P | 5V | 16MHz | 14 | 6 | 7(0-5, ADC) | 1 | 12 | 32 | Micro USB |
Seeeduino Lotus Cortex-M0+ | SAMD21 | 3.3V | 48MHz | 14 | 10 | 6 | 2 | 12 | 256 | Micro USB |
Seeeduino Cortex-M0+ | SAMD21 | 5V | 48MHz | 14 | 10 | 6 | 1 | 3 | 256 | Type-C |
Seeeduino Nano | Atmega328P | 5V | 16MHz | 14 | 6 | 8 | 1 | 1 | 32 | Type-C |
Seeeduino Crypto | ATmega4809 | 5V | 16Mhz | 14 | 5 | 6 | 1 | 3 | 48 | Type-C |
Seeeduino XIAO | SAMD21G18 | 3.3V | 48MHz | 11 | 10 | 11 | 1 | 0 | 256 |